SMTP
Generally speaking, when configuring SMTP (Simple Mail Transfer Protocol) settings for sending emails, whether for password recovery or any other purpose, it's crucial to ensure the settings are correctly specified. Here are some key points to include in documentation about using an SMTP configuration for a "forget password" library.
This code snippet sets up a utility for sending emails using nodemailer, a Node.js module for sending emails. Here's a detailed breakdown of each part of the code.
- - SMTP Server: Specify the address of the SMTP server you're using. This could be provided by your email service provider (e.g., smtp.gmail.com for Gmail).
- - Port Number: Different SMTP servers use different port numbers. Common ports include 25, 465 (SSL/TLS encrypted), and 587 (STARTTLS). Document which port your SMTP server requires.
- - Authentication: SMTP servers typically require authentication. Specify whether your SMTP server uses username/password authentication or other methods like NextAuth.
- - Encryption: It's crucial to use encryption (SSL/TLS) when transmitting sensitive information like passwords. Document whether your SMTP server requires SSL/TLS encryption.
- - From Address: Specify the email address that emails will be sent from. Ensure this matches the domain and configuration of your SMTP server to avoid issues like being flagged as spam.
- - Testing: Include steps to test the SMTP configuration to ensure emails are successfully sent. This might involve sending test emails or using tools like Telnet to verify connectivity.
Clear, step-by-step instructions will help users integrate SMTP effectively for their "forget password" functionality, ensuring reliable email delivery for password recovery emails.
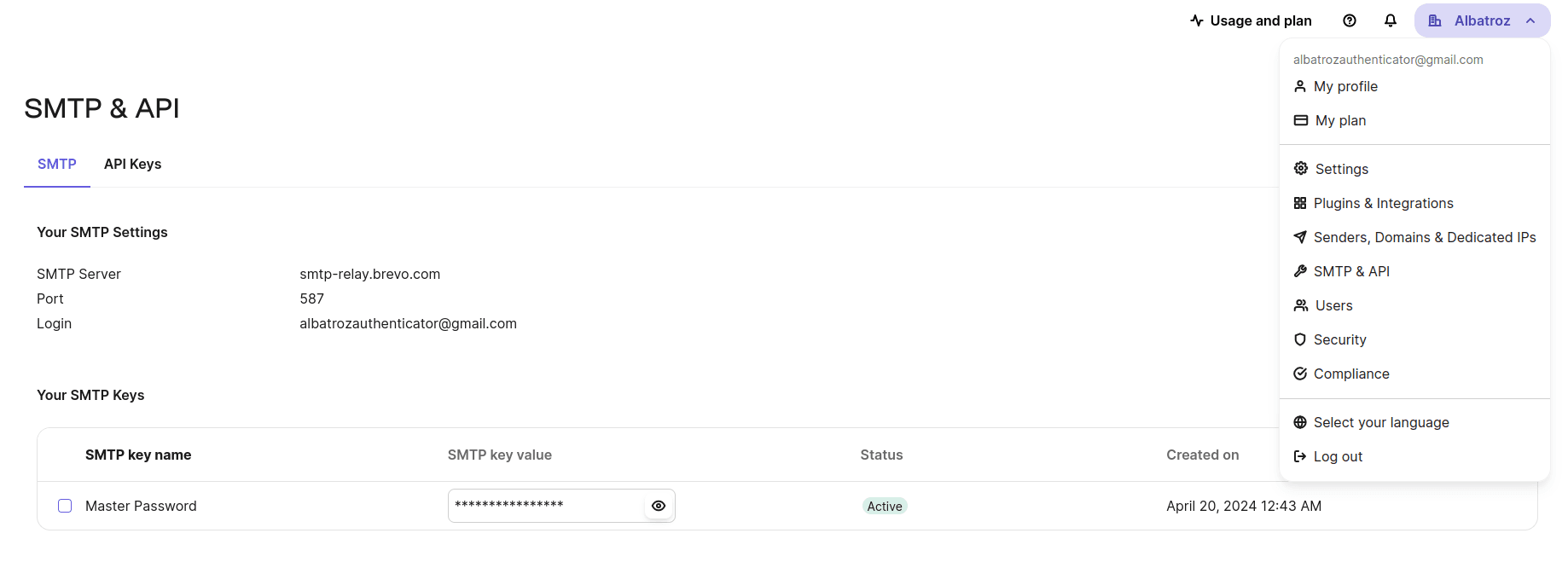
Transporter Configuration
./config/mail.ts
import nodemailer from "nodemailer";
import Env from '@/config/env'
export const transporter = nodemailer.createTransport({
host: Env.SMTP_HOST,
port: Number(Env.SMTP_PORT),
secure: false,
auth: {
user: Env.SMTP_USER,
pass: Env.SMTP_PASSWORD,
},
});
//TO send the email
export const sendEmail = async (
to: string,
subject: string,
html: string
): Promise<string | null> => {
const info = await transporter.sendMail({
from: Env.EMAIL_FROM,
to: to,
subject: subject,
html: html,
});
return info ?.messageId;
};
- - Creates a transporter object using nodemailer.createTransport.
- - Configures the SMTP server details:
- - host: The SMTP host address from the environment variable.
- - port: The SMTP port number, converted to a number using Number().
- - secure: Set to false, meaning the connection will not use SSL/TLS. This can be changed to true if SSL/TLS is required.
- - auth: Authentication details including the username and password from the environment variables.
sendEmail Function
- - sendEmail: An asynchronous function that sends an email.
- - Parameters:
- - to: The recipient's email address.
- - subject: The subject of the email.
- - html: The HTML content of the email.
- - transporter.sendMail: Uses the configured transporter to send the email with the specified from, to, subject, and html content.
- - The function returns the messageId of the sent email, which is useful for tracking or logging purposes. If messageId is not available, it returns null..
Summary
The code sets up an email sending utility using nodemailer. The transporter is configured using SMTP details from environment variables, ensuring sensitive information like SMTP credentials are not hardcoded in the codebase. The sendEmail function provides a convenient way to send emails with specified recipients, subjects, and HTML content, making it reusable throughout the application. The use of async/await ensures that the function handles asynchronous operations correctly.
Env class
This Env class is designed to retrieve and store environment variables used in the application. The environment variables are essential for configuration and are typically set outside of the codebase, such as in environment configuration files or through deployment settings. Here’s a detailed explanation of each part of the code:
./config/env.ts
class Env {
static SMTP_HOST: string = process.env.SMTP_HOST!;
static SMTP_PORT: string = process.env.SMTP_PORT!;
static SMTP_USER: string = process.env.SMTP_USER!;
static SMTP_PASSWORD: string = process.env.SMTP_PASSWORD!;
static SMTP_SECURE: string = process.env.SMTP_SECURE!;
static EMAIL_FROM: string = process.env.EMAIL_FROM!;
static SECRET_KEY: string = process.env.NEXTAUTH_SECRET!;
static APP_URL: string = process.env.APP_URL!;
}
export default Env
Static Properties
- - Declares static properties for the class.
- - Each property corresponds to an environment variable.
- - The process.env object is used to access the environment variables.
- - The exclamation mark (!) at the end of each assignment tells TypeScript that these variables are expected to be non-null and non-undefined (type assertion).
SMTP Configuration:
- - These properties store the SMTP (Simple Mail Transfer Protocol) server configuration details:
- - SMTP_HOST: The host address of the SMTP server.
- - SMTP_PORT: The port number to connect to the SMTP server.
- - SMTP_USER: The username for SMTP authentication.
- - SMTP_PASSWORD: The password for SMTP authentication.
- - SMTP_SECURE: Whether to use a secure connection (true/false).
Email Configuration:
- - EMAIL_FROM: The default email address used in the "From" field when sending emails.
Application Configuration:
- - SECRET_KEY: The secret key used for encryption and other secure operations (from NEXTAUTH_SECRET environment variable).
- - APP_URL: The base URL of the application.
Summary
The Env class encapsulates the retrieval of environment variables, providing a convenient and organized way to access these values throughout the application. This approach helps maintain a clear separation between configuration and code, making the application more flexible and easier to manage across different environments (development, testing, production). The use of static properties means that these configuration values can be accessed directly through the class without needing to instantiate it.